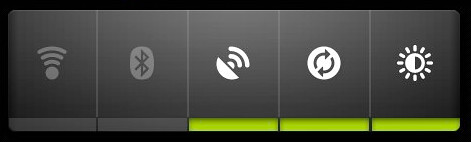
When we plan to provide widget in our own application, an important thing to think about is the communication model between the widget and application. In a simiplified manner, the commucation model is shown below.

The widget is shown on home screen(which is a AppWidgetHost), and user can interact(e.g., touch) with the widget. The result of the interaction is either showing an activity to the user to display more information, or controlling the state of a background service. Meanwhile, the background service may proactively update the widget to inform user current state. The communication model is bidirectional.
Launch activity from widget
To launch an activity from widget, we can use RemoteViews's setOnClickPendingIntent method to set a intent for the target button. Once the button is clicked, the intent will be sent to start desired activity. The snippet below shows how to do this in AppWidgetProvider.
1 import android.app.PendingIntent;
2
3 import android.appwidget.AppWidgetManager;
4 import android.appwidget.AppWidgetProvider;
5
6 import android.content.Context;
7 import android.content.Intent;
8
9 import android.widget.RemoteViews;
10
11 public class GestureAppWidgetProvider extends AppWidgetProvider {
12
13 public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
14 final int N = appWidgetIds.length;
15
16 // Perform this loop procedure for each App Widget that belongs to this provider
17 for (int i=0; i<N; i++) {
18 int appWidgetId = appWidgetIds[i];
19
20 // Create an Intent to launch Activity
21 Intent intent = new Intent(context, MainActivity.class);
22 PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, 0);
23
24 // Get the layout for the App Widget and attach an on-click listener
25 // to the button
26 RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.appwidget);
27 views.setTextColor(R.id.startRecord, Color.GREEN);
28 views.setOnClickPendingIntent(R.id.startRecord, pendingIntent);
29
30 // Tell the AppWidgetManager to perform an update on the current app widget
31 appWidgetManager.updateAppWidget(appWidgetId, views);
32 }
33 }
34 }
2
3 import android.appwidget.AppWidgetManager;
4 import android.appwidget.AppWidgetProvider;
5
6 import android.content.Context;
7 import android.content.Intent;
8
9 import android.widget.RemoteViews;
10
11 public class GestureAppWidgetProvider extends AppWidgetProvider {
12
13 public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
14 final int N = appWidgetIds.length;
15
16 // Perform this loop procedure for each App Widget that belongs to this provider
17 for (int i=0; i<N; i++) {
18 int appWidgetId = appWidgetIds[i];
19
20 // Create an Intent to launch Activity
21 Intent intent = new Intent(context, MainActivity.class);
22 PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, 0);
23
24 // Get the layout for the App Widget and attach an on-click listener
25 // to the button
26 RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.appwidget);
27 views.setTextColor(R.id.startRecord, Color.GREEN);
28 views.setOnClickPendingIntent(R.id.startRecord, pendingIntent);
29
30 // Tell the AppWidgetManager to perform an update on the current app widget
31 appWidgetManager.updateAppWidget(appWidgetId, views);
32 }
33 }
34 }
Send message to service from widget
The skeleton of code to send message to a service is pretty much the same as the snippet above. The change we need to make is substitute PendingIntent.getActivity with PendingIntent.getBroadCast. The result is once we clicked the button, a broadcast Intent will be sent, and our AppWidgetProvider(which is a subclass of BroadcastReceiver) will get this intent. Thie AppWidgetProvider runs in our application's process, so it can send the message to our service with StartService.
1 public class WeatherWidgetProvider extends AppWidgetProvider {
2 public static String REFRESH_ACTION = "com.example.android.weatherlistwidget.REFRESH";
3
4 @Override
5 public void onReceive(Context ctx, Intent intent) {
6 final String action = intent.getAction();
7 if (action.equals(REFRESH_ACTION)) {
8 // send message to background service via startService here
9 // ..............
10 }
11 super.onReceive(ctx, intent);
12 }
13
14 @Override
15 public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
16 for (int i = 0; i < appWidgetIds.length; ++i) {
17 final RemoteViews rv = new RemoteViews(context.getPackageName(), R.layout.widget_layout);
18 rv.setRemoteAdapter(appWidgetIds[i], R.id.weather_list, intent);
19
20 // Set the empty view to be displayed if the collection is empty. It must be a sibling
21 // view of the collection view.
22 rv.setEmptyView(R.id.weather_list, R.id.empty_view);
23
24 // Bind the click intent for the refresh button on the widget
25 final Intent refreshIntent = new Intent(context, WeatherWidgetProvider.class);
26 refreshIntent.setAction(WeatherWidgetProvider.REFRESH_ACTION);
27 final PendingIntent refreshPendingIntent = PendingIntent.getBroadcast(context, 0,
28 refreshIntent, PendingIntent.FLAG_UPDATE_CURRENT);
29 rv.setOnClickPendingIntent(R.id.refresh, refreshPendingIntent);
30
31 appWidgetManager.updateAppWidget(appWidgetIds[i], rv);
32 }
33 super.onUpdate(context, appWidgetManager, appWidgetIds);
34 }
35 }
2 public static String REFRESH_ACTION = "com.example.android.weatherlistwidget.REFRESH";
3
4 @Override
5 public void onReceive(Context ctx, Intent intent) {
6 final String action = intent.getAction();
7 if (action.equals(REFRESH_ACTION)) {
8 // send message to background service via startService here
9 // ..............
10 }
11 super.onReceive(ctx, intent);
12 }
13
14 @Override
15 public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
16 for (int i = 0; i < appWidgetIds.length; ++i) {
17 final RemoteViews rv = new RemoteViews(context.getPackageName(), R.layout.widget_layout);
18 rv.setRemoteAdapter(appWidgetIds[i], R.id.weather_list, intent);
19
20 // Set the empty view to be displayed if the collection is empty. It must be a sibling
21 // view of the collection view.
22 rv.setEmptyView(R.id.weather_list, R.id.empty_view);
23
24 // Bind the click intent for the refresh button on the widget
25 final Intent refreshIntent = new Intent(context, WeatherWidgetProvider.class);
26 refreshIntent.setAction(WeatherWidgetProvider.REFRESH_ACTION);
27 final PendingIntent refreshPendingIntent = PendingIntent.getBroadcast(context, 0,
28 refreshIntent, PendingIntent.FLAG_UPDATE_CURRENT);
29 rv.setOnClickPendingIntent(R.id.refresh, refreshPendingIntent);
30
31 appWidgetManager.updateAppWidget(appWidgetIds[i], rv);
32 }
33 super.onUpdate(context, appWidgetManager, appWidgetIds);
34 }
35 }
Update widget from service
To update a widget from service, we can send a broadcast message from the background service to AppWidgetProvider. Once the AppWidgetProvider receives the message, it tries to fetch current state and calls notifyAppWidgetViewDataChanged function to refresh the widget.
1 public void onReceive(Context ctx, Intent intent) {
2 final String action = intent.getAction();
3 if (action.equals(SHOW_NEW_DATA_ACTION)) {
4 final AppWidgetManager mgr = AppWidgetManager.getInstance(context);
5 final ComponentName cn = new ComponentName(context, WeatherWidgetProvider.class);
6 mgr.notifyAppWidgetViewDataChanged(mgr.getAppWidgetIds(cn), R.id.weather_list);
7 }
8 super.onReceive(ctx, intent);
9 }
2 final String action = intent.getAction();
3 if (action.equals(SHOW_NEW_DATA_ACTION)) {
4 final AppWidgetManager mgr = AppWidgetManager.getInstance(context);
5 final ComponentName cn = new ComponentName(context, WeatherWidgetProvider.class);
6 mgr.notifyAppWidgetViewDataChanged(mgr.getAppWidgetIds(cn), R.id.weather_list);
7 }
8 super.onReceive(ctx, intent);
9 }
Reference:
android WeatherListWidget sampleIntroducing home screen widgets and the AppWidget framework
android appwidget source code
android appwidget service source code