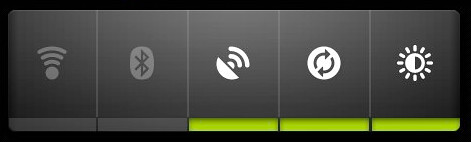
When we plan to provide widget in our own application, an important thing to think about is the communication model between the widget and application. In a simiplified manner, the commucation model is shown below.

The widget is shown on home screen(which is a AppWidgetHost), and user can interact(e.g., touch) with the widget. The result of the interaction is either showing an activity to the user to display more information, or controlling the state of a background service. Meanwhile, the background service may proactively update the widget to inform user current state. The communication model is bidirectional.
Launch activity from widget
To launch an activity from widget, we can use RemoteViews's setOnClickPendingIntent method to set a intent for the target button. Once the button is clicked, the intent will be sent to start desired activity. The snippet below shows how to do this in AppWidgetProvider.
1 import android.app.PendingIntent;
2
3 import android.appwidget.AppWidgetManager;
4 import android.appwidget.AppWidgetProvider;
5
6 import android.content.Context;
7 import android.content.Intent;
8
9 import android.widget.RemoteViews;
10
11 public class GestureAppWidgetProvider extends AppWidgetProvider {
12
13 public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
14 final int N = appWidgetIds.length;
15
16 // Perform this loop procedure for each App Widget that belongs to this provider
17 for (int i=0; i<N; i++) {
18 int appWidgetId = appWidgetIds[i];
19
20 // Create an Intent to launch Activity
21 Intent intent = new Intent(context, MainActivity.class);
22 PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, 0);
23
24 // Get the layout for the App Widget and attach an on-click listener
25 // to the button
26 RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.appwidget);
27 views.setTextColor(R.id.startRecord, Color.GREEN);
28 views.setOnClickPendingIntent(R.id.startRecord, pendingIntent);
29
30 // Tell the AppWidgetManager to perform an update on the current app widget
31 appWidgetManager.updateAppWidget(appWidgetId, views);
32 }
33 }
34 }
2
3 import android.appwidget.AppWidgetManager;
4 import android.appwidget.AppWidgetProvider;
5
6 import android.content.Context;
7 import android.content.Intent;
8
9 import android.widget.RemoteViews;
10
11 public class GestureAppWidgetProvider extends AppWidgetProvider {
12
13 public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
14 final int N = appWidgetIds.length;
15
16 // Perform this loop procedure for each App Widget that belongs to this provider
17 for (int i=0; i<N; i++) {
18 int appWidgetId = appWidgetIds[i];
19
20 // Create an Intent to launch Activity
21 Intent intent = new Intent(context, MainActivity.class);
22 PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, 0);
23
24 // Get the layout for the App Widget and attach an on-click listener
25 // to the button
26 RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.appwidget);
27 views.setTextColor(R.id.startRecord, Color.GREEN);
28 views.setOnClickPendingIntent(R.id.startRecord, pendingIntent);
29
30 // Tell the AppWidgetManager to perform an update on the current app widget
31 appWidgetManager.updateAppWidget(appWidgetId, views);
32 }
33 }
34 }
Send message to service from widget
The skeleton of code to send message to a service is pretty much the same as the snippet above. The change we need to make is substitute PendingIntent.getActivity with PendingIntent.getBroadCast. The result is once we clicked the button, a broadcast Intent will be sent, and our AppWidgetProvider(which is a subclass of BroadcastReceiver) will get this intent. Thie AppWidgetProvider runs in our application's process, so it can send the message to our service with StartService.
1 public class WeatherWidgetProvider extends AppWidgetProvider {
2 public static String REFRESH_ACTION = "com.example.android.weatherlistwidget.REFRESH";
3
4 @Override
5 public void onReceive(Context ctx, Intent intent) {
6 final String action = intent.getAction();
7 if (action.equals(REFRESH_ACTION)) {
8 // send message to background service via startService here
9 // ..............
10 }
11 super.onReceive(ctx, intent);
12 }
13
14 @Override
15 public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
16 for (int i = 0; i < appWidgetIds.length; ++i) {
17 final RemoteViews rv = new RemoteViews(context.getPackageName(), R.layout.widget_layout);
18 rv.setRemoteAdapter(appWidgetIds[i], R.id.weather_list, intent);
19
20 // Set the empty view to be displayed if the collection is empty. It must be a sibling
21 // view of the collection view.
22 rv.setEmptyView(R.id.weather_list, R.id.empty_view);
23
24 // Bind the click intent for the refresh button on the widget
25 final Intent refreshIntent = new Intent(context, WeatherWidgetProvider.class);
26 refreshIntent.setAction(WeatherWidgetProvider.REFRESH_ACTION);
27 final PendingIntent refreshPendingIntent = PendingIntent.getBroadcast(context, 0,
28 refreshIntent, PendingIntent.FLAG_UPDATE_CURRENT);
29 rv.setOnClickPendingIntent(R.id.refresh, refreshPendingIntent);
30
31 appWidgetManager.updateAppWidget(appWidgetIds[i], rv);
32 }
33 super.onUpdate(context, appWidgetManager, appWidgetIds);
34 }
35 }
2 public static String REFRESH_ACTION = "com.example.android.weatherlistwidget.REFRESH";
3
4 @Override
5 public void onReceive(Context ctx, Intent intent) {
6 final String action = intent.getAction();
7 if (action.equals(REFRESH_ACTION)) {
8 // send message to background service via startService here
9 // ..............
10 }
11 super.onReceive(ctx, intent);
12 }
13
14 @Override
15 public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) {
16 for (int i = 0; i < appWidgetIds.length; ++i) {
17 final RemoteViews rv = new RemoteViews(context.getPackageName(), R.layout.widget_layout);
18 rv.setRemoteAdapter(appWidgetIds[i], R.id.weather_list, intent);
19
20 // Set the empty view to be displayed if the collection is empty. It must be a sibling
21 // view of the collection view.
22 rv.setEmptyView(R.id.weather_list, R.id.empty_view);
23
24 // Bind the click intent for the refresh button on the widget
25 final Intent refreshIntent = new Intent(context, WeatherWidgetProvider.class);
26 refreshIntent.setAction(WeatherWidgetProvider.REFRESH_ACTION);
27 final PendingIntent refreshPendingIntent = PendingIntent.getBroadcast(context, 0,
28 refreshIntent, PendingIntent.FLAG_UPDATE_CURRENT);
29 rv.setOnClickPendingIntent(R.id.refresh, refreshPendingIntent);
30
31 appWidgetManager.updateAppWidget(appWidgetIds[i], rv);
32 }
33 super.onUpdate(context, appWidgetManager, appWidgetIds);
34 }
35 }
Update widget from service
To update a widget from service, we can send a broadcast message from the background service to AppWidgetProvider. Once the AppWidgetProvider receives the message, it tries to fetch current state and calls notifyAppWidgetViewDataChanged function to refresh the widget.
1 public void onReceive(Context ctx, Intent intent) {
2 final String action = intent.getAction();
3 if (action.equals(SHOW_NEW_DATA_ACTION)) {
4 final AppWidgetManager mgr = AppWidgetManager.getInstance(context);
5 final ComponentName cn = new ComponentName(context, WeatherWidgetProvider.class);
6 mgr.notifyAppWidgetViewDataChanged(mgr.getAppWidgetIds(cn), R.id.weather_list);
7 }
8 super.onReceive(ctx, intent);
9 }
2 final String action = intent.getAction();
3 if (action.equals(SHOW_NEW_DATA_ACTION)) {
4 final AppWidgetManager mgr = AppWidgetManager.getInstance(context);
5 final ComponentName cn = new ComponentName(context, WeatherWidgetProvider.class);
6 mgr.notifyAppWidgetViewDataChanged(mgr.getAppWidgetIds(cn), R.id.weather_list);
7 }
8 super.onReceive(ctx, intent);
9 }
Reference:
android WeatherListWidget sampleIntroducing home screen widgets and the AppWidget framework
android appwidget source code
android appwidget service source code
171 comments:
in sending message, how to send broadcast message in other class the onreceive is on the fragment class is this possible ?
Very informative ..i suggest this blog to my friends..Thank you for sharingAndroid Training
The knowledge of technology you have been sharing thorough this post is very much helpful to develop new idea. here by i also want to share this.
rpa Training in annanagar
blue prism Training in annanagar
automation anywhere Training in annanagar
iot Training in annanagar
rpa Training in marathahalli
blue prism Training in marathahalli
automation anywhere Training in marathahalli
blue prism training in jayanagar
automation anywhere training in jayanagar
Inspiring writings and I greatly admired what you have to say , I hope you continue to provide new ideas for us all and greetings success always for you..Keep update more information..
rpa Training in Chennai
rpa Training in bangalore
rpa Training in pune
blueprism Training in Chennai
blueprism Training in bangalore
blueprism Training in pune
rpa online training
Great post! I am actually getting ready to across this information, It’s very helpful for this blog.Also great with all of the valuable information you have Keep up the good work you are doing well.
automation anywhere training in chennai
automation anywhere training in bangalore
automation anywhere training in pune
automation anywhere online training
blueprism online training
rpa Training in sholinganallur
rpa Training in annanagar
iot-training-in-chennai
blueprism-training-in-pune
automation-anywhere-training-in-pune
All are saying the same thing repeatedly, but in your blog I had a chance to get some useful and unique information, I love your writing style very much, I would like to suggest your blog in my dude circle, so keep on updates.
automation anywhere training in chennai
automation anywhere training in bangalore
automation anywhere training in pune
automation anywhere online training
blueprism online training
rpa Training in sholinganallur
rpa Training in annanagar
iot-training-in-chennai
blueprism-training-in-pune
automation-anywhere-training-in-pune
The knowledge of technology you have been sharing thorough this post is very much helpful to develop new idea. here by i also want to share this.
Click here:
angularjs training in sholinganallur
Click here:
angularjs training in btm
Wonderful bloggers like yourself who would positively reply encouraged me to be more open and engaging in commenting.So know it's helpful.
Data Science Training in Chennai
Data science training in bangalore
Data science online training
Data science training in pune
Data science training in kalyan nagar
selenium training in chennai
Thanks for posting this info. I just want to let you know that I just check out your site and I find it very interesting and informative. I can't wait to read lots of your posts
java training in chennai | java training in bangalore
java online training | java training in pune
java training in chennai | java training in bangalore
It is very useful to me. Keep sharing this kind of useful information.
IoT Certification | Internet of Things Training in Chennai
I am so proud of you and your efforts and work make me realize that anything can be done with patience and sincerity. Well, I am here to say that your work has inspired me without a doubt.
python training in chennai
python training in Bangalore
Needed to compose you a very little word to thank you yet again regarding the nice suggestions you’ve contributed here.
Blueprism training in btm
Blueprism online training
This is a terrific article, and that I would really like additional info if you have got any. I’m fascinated with this subject and your post has been one among the simplest I actually have read.
angularjs Training in bangalore
angularjs Training in btm
angularjs Training in electronic-city
angularjs online Training
angularjs Training in marathahalli
Informative post, thanks for taking time to share this page.
Robotic Process Automation Training in Chennai
Robotic Process Automation Certification
RPA Training in Chennai
AWS Training in Chennai
Angularjs Training in Chennai
ccna Training in Chennai
I am really happy with your blog because your article is very unique and powerful for new reader.
Click here:
selenium training in chennai
selenium training in bangalore
selenium training in Pune
selenium training in pune
Selenium Online Training
https://rpeszek.blogspot.com/2014/10/i-dont-like-hibernategrails-part-11_31.html
Interesting Post. I liked your style of writing. It is very unique. Thanks for Posting.
Node JS Training in Chennai
Node JS Course in Chennai
Node JS Advanced Training
Node JS Training Institute in chennai
Node JS Training Institutes in chennai
Node JS Course
Excellent way of expressing your ideas with a clear vision, Keep updating.
Selenium Training in Chennai
Selenium Training
iOS Training in Chennai
Digital Marketing Training in Chennai
core java training in chennai
PHP Training in Chennai
PHP Course in Chennai
Excellent post! keep sharing such a post
Guest posting sites
Education
This is really too useful and have more ideas and keep sharing many techniques. Eagerly waiting for your new blog keep doing more.
Aws Training in Bangalore
Aws Course in Bangalore
Best Aws Training in Bangalore
hadoop classes in bangalore
hadoop institute in bangalore
Aws Training Institutes in Bangalore
Aws Certification Training in Bangalore
It is very excellent blog and useful article thank you for sharing with us, keep posting.
Primavera Training
Primavera p6 Training
Primavera Training Institute in Chennai
Primavera Training Institutes in Chennai
Nice post. I learned some new information. Thanks for sharing.
Xamarin Training in Chennai
Xamarin Course in Chennai
Xamarin Training
Xamarin Course
Xamarin Training Course
Xamarin Classes
Best Xamarin Course
Thanks for sharing such an amazing post. Your style of writing is very unique. It made me mesmerized in your words. Keep on writing.
Informatica Training in Chennai
Informatica Training Center Chennai
Best Informatica Training in Chennai
Informatica course in Chennai
Informatica Training center in Chennai
Informatica Training
Learn Informatica
Informatica course
Thank you for such an informative post. Thank you for sharing with us.
Spark Training Academy Chennai
Apache Spark Training
Spark Training Institute in Adyar
Spark Training Institute in Velachery
Spark Training Institute in Tambaram
Awesome Post . Your way of expressing things makes reading very enjoyable. Thanks for posting.
Ethical Hacking Course in Chennai
Hacking Course in Chennai
Ethical Hacking Training in Chennai
Certified Ethical Hacking Course in Chennai
Ethical Hacking Course
Ethical Hacking Certification
IELTS coaching in Chennai
IELTS Training in Chennai
Thanks for the wonderful work. It is really superbb...
Selenium Training in Chennai
Selenium Training
iOS Training in Chennai
French Classes in Chennai
Big Data Training in Chennai
Loadrunner Training in Chennai
Loadrunner Training
You are an awesome writer. The way you deliver is exquisite. Pls keep up your work.
Spoken English Classes in Chennai
Best Spoken English Classes in Chennai
Spoken English Class in Chennai
Spoken English in Chennai
Best Spoken English Class in Chennai
English Coaching Classes in Chennai
Best Spoken English Institute in Chennai
Nice informative post...Thanks for sharing..
Article submission sites
Guest posting sites
Thanks for sharing this informative post. Regards.
Microsoft Dynamics CRM Training in Chennai | Microsoft Dynamics Training in Chennai | Microsoft Dynamics CRM Training | Microsoft Dynamics CRM Training institutes in Chennai | Microsoft Dynamics Training | Microsoft CRM Training | Microsoft Dynamics CRM Training Courses | CRM Training in Chennai
Thanks for the author very nice to read
php training course in chennai
Excellent Article. Thanks Admin
DevOps Training in Chennai
Cloud Computing Training in Chennai
IT Software Training in Chennai
This is really impressive post, I am inspired with your post, do post more blogs like this, I am waiting for your blogs.
Regards,
Devops Training in Chennai | Devops Certification in Chennai
Awesome blog if our training additional way as an SQL and PL/SQL trained as individual, you will be able to understand other applications more quickly and continue to build your skill set which will assist you in getting hi-tech industry jobs as possible in future courese of action
Devops Training in Chennai | Devops Training Institute in Chennai
I have been meaning to write something like this on my website and you have given me an idea. Cheers.
devops online training
aws online training
data science with python online training
data science online training
rpa online training
We are a group of volunteers and starting a new initiative in a community. Your blog provided us valuable information to work on.You have done a marvellous job!
Microsoft Azure online training
Selenium online training
Java online training
Python online training
uipath online training
Fuck you dickhead
THis post is awesome. You have really made my day. inspirational gym quotes
Nice information, valuable and excellent article, lots of great information, thanks for sharing with peoples.
ExcelR Data Science Course in Bangalore
Glad to chat your blog, I seem to be forward to more reliable articles and I think we all wish to thank so many good articles, blog to share with us.
BIG DATA COURSE MALAYSIA
Thanks for the nice blog. It was very useful for me. I'm happy I found this blog. Thank you for sharing with us,I too always learn something new from your post.
machine learning course malaysia
Nice blog, it's so knowledgeable, informative, and good looking site. I appreciate your hard work. Good job. Thank you for this wonderful sharing with us. Keep Sharing.
Kindly visit us @ 100% Job Placement | Best Colleges for Computer Engineering
Biomedical Engineering Colleges in Coimbatore | Best Biotechnology Colleges in Tamilnadu
Biotechnology Colleges in Coimbatore | Biotechnology Courses in Coimbatore
Best MCA Colleges in Tamilnadu | Best MBA Colleges in Coimbatore
Engineering Courses in Tamilnadu | Engg Colleges in Coimbatore
یک مقاله جالب و جالب. با تشکر برای به اشتراک گذاری
lều xông hơi mini
mua lều xông hơi ở đâu
lều xông hơi gia đình
bán lều xông hơi
xông hơi hồng ngoại
It should be noted that whilst ordering papers for sale at paper writing service, you can get unkind attitude. In case you feel that the bureau is trying to cheat you, don't buy term paper from it. Love it.
Just seen your Article, it amazed me and surpised me with god thoughts that eveyone will benefit from it. It is really a very informative post for all those budding entreprenuers planning to take advantage of post for business expansions. You always share such a wonderful articlewhich helps us to gain knowledge .Thanks for sharing such a wonderful article, It will be deinitely helpful and fruitful article.
Thanks
DedicatedHosting4u.com
It has fully emerged to crown Singapore's southern shores and undoubtedly placed her on the global map of residential landmarks. I still scored the more points than I ever have in a season for GS. I think you would be hard pressed to find somebody with the same consistency I have had over the years so I am happy with that.
big data course malaysia
Useful information
Top 5 best PPSSPP games for Android
Top 6 Best free online video convertor websites
Bill Gates admits losing to android as his biggest mistake
Top 9 best free tools and website to convert speech into text online
Advantages of choosing proper Antivirus for your PC
Top 8 Reasons Why Government Will Be Slow to Accept the Cloud
Things to be kept in mind while choosing a recovery software
Android vs. Other mobile Operating system and how google is best
Iamrjrahul WR3D 2K17
Poke Meaning In Hindi Poke ka Matlab Kya Hai
UltraTech4You
Get Into PC
Earn Paytm Cash By Watching Ads ₹10,000/Month
Parvez Alam
Coin master is one of the famous and grossing games right now. you can spin the wheel and believe in fortune to get a lot of Spins. But you end up getting small amounts of spins. For those who are searching for Coin Master unlimited spins, this article is very useful to them. https://meditips.in/coin-master-free-spins/
Poweramp is a powerful music player for Android. Using Poweramp Full Version Unlocker [Cracker] For Android, you can access the extra features of the Poweramp music player. https://www.hightechpicker.com/2019/08/poweramp-full-version-unlocker.html
In our culture, the practice of treatment through various burn fat herbs and
spices is widely prevalent. This is mainly due to the reason that different burn fat herbs grow in great abundance here. In addition to the
treatment of various ailments these herbs prove beneficial in Healthy Ways To Lose Weight
, especially for those who want to burn fat herbs
we live in a world where diseases and their prevalence has gone off
the charts. With the ever-growing incidences of illnesses and
sufferings, one finds themselves caught up in a loop of medications
and doctors’ visits. We, at https://goodbyedoctor.com/ , aim to find solutions for
all your health-related problems in the most natural and harmless ways.
We’re a website dedicated to providing you with the best of home
remedies, organic solutions, and show you a path towards a healthy,
happy life. visit https://goodbyedoctor.com/
this site daily to know more about health tips and beauty tips.
Nice article
Thanks for sharing the information
Please visit the leadmirror to know your SEO position on the google.
What's new in PUBG Mobile Season 9 Update - PUBG Mobile Update v 14.5
amazing post written ... It shows your effort and dedication. Thanks for share such a nice post. Please check whatsapp status in hindi and best wifi names
Thanks for providing the updated information cyber security online training hyderbad
Hacking course online is to learn a professional hacking course
Keep posting
interview-questions/aptitude/permutation-and-combination/how-many-groups-of-6-
persons-can-be-formed
tutorials/oracle/oracle-delete
technology/chrome-flags-complete-guide-enhance-browsing-experience/
interview-questions/aptitude/time-and-work/a-alone-can-do-1-4-of-the-work-in-2-days
interview-questions/programming/recursion-and-iteration/integer-a-40-b-35-c-20-d-10
-comment-about-the-output-of-the-following-two-statements
tutorials/apache-pig/apache-pig-subtract-function
errors-and-fixes/csharp/xml-serializer-there-was-an-error-reflecting-type
interview-questions/aptitude/simple-interest/if-the-simple-interest-on-a-certain-sum-
of-money
interview-questions/aptitude/compound-interest/rs-5887-is-divided-between-shyam-
and-ram
letters/tag/specimen-presentation-of-letters-issued-by-company/
a alone can do 14 of the work in 2 days
Rs 5887 is divided between shyam and ram
If the simple interest on a certain sum...
xml serializer...
Apache pig subtract function...
Integer a 40 b 35 c 20 d 10...
How many groups of 6 persons...
Chrome flags complete guide...
Oracle delete..
specimen presentation of letters..
I found your article on Google when I was surfing, it is written very nicely and is optimized .Thank you I visit your website regularly.
download ncert books
Very good post, keep sending us such informative articles I visit your website on a regular basis.
affairscloud current affairs quiz
thanks for share this amazing article 먹튀검증커뮤니티
This is really an awesome post, thanks for it. Keep adding more information to this.selenium training in bangalore
Your articles really impressed for me,because of all information so nice.manual testing training in bangalore
Nice information, you write very nice articles, I visit your website for regular updates.
haircut for girls
I Love your website i am ready your all website article 먹튀검증
I found your article on Google when I was surfing, it is written very nicely and is optimized .Thank you I visit your website regularly.
software free download full version
PhenQ Reviews - Is PhenQ a new Scam?
Does it really work? Read this honest review and make a wise purchase decision. PhenQ ingredients are natural and ...
It has been deemed fit for use in the market. It is not found to be a Scam weight loss pill.
By far it is the safest and most effective weight loss pill available in the market today.
Phenq reviews ..This is a powerful slimming formula made by combining the multiple weight loss
benefits of various PhenQ ingredients. All these are conveniently contained in one pill. It helps you get the kind of body that you need. The ingredients of
the pill are from natural sources so you don’t have to worry much about the side effects that come with other types of dieting pills.Is PhenQ safe ? yes this is completly safe.
Where to buy PhenQ ? you can order online. you don`t know Where to order phenq check this site .
visit https://mpho.org/ this site to know more about PhenQ Reviews.
Its really helpful for the users of this site. I am also searching about these type of sites now a days. So your site really helps me for searching the new and great stuff.
blue prism training in bangalore
blue prism courses in bangalore
blue prism classes in bangalore
blue prism training institute in bangalore
blue prism course syllabus
best blue prism training
blue prism training centers
It is very good and useful for students and developer.Learned a lot of new things from your post Good creation,thanks for give a good information.
robotic process automation (rpa) training in bangalore
robotic process automation (rpa) courses in bangalore
robotic process automation (rpa) classes in bangalore
robotic process automation (rpa) training institute in bangalore
robotic process automation (rpa) course syllabus
best robotic process automation (rpa) training
robotic process automation (rpa) training centers
Excellent post for the people who really need information for this technology.
uipath training in bangalore
uipath courses in bangalore
uipath classes in bangalore
uipath training institute in bangalore
uipath course syllabus
best uipath training
uipath training centers
Being new to the blogging world I feel like there is still so much to learn. Your tips helped to clarify a few things for me as well as giving.
openspan training in bangalore
openspan courses in bangalore
openspan classes in bangalore
openspan training institute in bangalore
openspan course syllabus
best openspan training
openspan training centers
Really i appreciate the effort you made to share the knowledge. The topic here i found was really effective...
Learn Best PEGA Training in Bangalore from Experts. Softgen Infotech offers the Best PegaTraining in Bangalore.100% Placement Assistance, Live Classroom Sessions, Only Technical Profiles, 24x7 Lab Infrastructure Support.
very nice
inplant training in chennai
inplant training in chennai
inplant training in chennai for it.php
Bermuda web hosting
Botswana hosting
armenia web hosting
dominican republic web hosting
iran hosting
palestinian territory web hosting
iceland web hosting
nice
inplant training in chennai
inplant training in chennai for it
suden web hosting
tunisia hosting
uruguay web hosting
Bermuda web hosting
Botswana hosting
armenia web hosting
lebanon web hosting
nice
inplant training in chennai
inplant training in chennai
online python internship
online web design
online machine learning internship
online internet of things internship
online cloud computing internship
online Robotics
online penetration testing
파워볼사이트
The article was up to the point and described the information very effectively. Thanks to blog author for wonderful and informative post
start learning digital marketing
I am really enjoying reading your well written articles. It looks like you spend a lot of effort and time on your blog. I have bookmarked it and I am looking forward to reading new articles. Keep up the good work.
ai training in bangalore
Machine Learning Training in Bangalore
Truly, this article is really one of the very best in the history of articles. I am a antique ’Article’ collector and I sometimes read some new articles if I find them interesting. And I found this one pretty fascinating and it should go into my collection. Very good work!
data science training in bangalore
data science interview questions
http://greenhomesgroup.com/- A 16+ year old Creative Media
Solutions company.
Engaged in Practical Creativity Advertising agencies in chennai for its clients from India,Europe and the US.
A proven portfolio of work across diverseTop Graphic design studios in chennai media for its clients from different domains.
An intricate3D augmented reality fusion of insightful strategy, cutting-edgeBranding agency in chennai
ideas, and intelligent media integration is what we callCorporate Film Makers in chennai practical creativity.
Check Our Website http://greenhomesgroup.com/.
Thisblogtruly has all the info I wanted concerning this subject and didn’t know who to ask.
thanx for sharing the quality post.
ccc exam paper 2020
Gold and silver for life reviews.
Thousands Across The Globe Using Mineshaft Bhindari gold and silver for life Training To Protect Their Wealth And Creating A Passive Income of 12% To 26.4% Per Year….
Gold and silver for life reviews- How It Works?
Minesh Bhindi created Gold and silver for life reviews because, after a long career in helping people grow their wealth through investment,
he noticed something that he felt should benefit everyone. Since 2010, Gold and Silver for life has been helping people grow their wealth securely through strategic Investing in precious metals , gold and silver.
As proud founder of Reverent Capital, a secure investment advisory firm, he consults with high net worth individuals from around the globe on the importance of secure
investments in gold and silver
Learn How to invest in gold from here kingsslyn.com now.
Whatever we gathered information from the blogs, we should implement that in practically then only we can understand that exact thing clearly cyber security training courses , but it’s no need to do it, because you have explained the concepts very well. It was crystal clear, keep sharing..
Hey,
Uselessly I am not Commenting on to the post But when I Saw your post It was Amazing. It any News you want to know National New Today
The TrendyFeed
Latest New Today
Technology New Today
Thanks,
The TrendyFeed
Thank you for sharing valuable information. Thanks for providing a great informatic blog, really nice required information & the things I never imagined. Thanks you once again Marvel Future Fight Mod Apk
Nice blog...
Best Travels in Madurai | Tours and Travels in Madurai | Best tour operators in Madurai
HI
Are you Looking For Digital Marketing In Noida. We have Team of expert for Digital marketing internship with 100% placementBest Digital marketing Agnecy In Noida
Nice and Great WEbsite thank you for share this amazing website 구글상위대행
Thanks for sharing.
Data Science Course
Data Science Course in Marathahalli
Data Science Course Training in Bangalore
Wow What a Nice and Great Article, Thank You So Much for Giving Us Such a Nice & Helpful Information, please keep writing and publishing these types of helpful articles, I visit your website regularly.
bharatpur pin code
good Congratulations guys, quality information you have given!!!..Its really useful blog. Thanks for sharing this useful 구글상위노출
You might comment on the order system of the blog. You should chat it's splendid. Your blog audit would swell up your visitors. I was very pleased to find this site.I wanted to thank you for this great read!!
machine learning course in pune
crowdsourcehttp://www.incruiter.com recruitment agency.
We ’incruiter’ provide a uniquerecruitment agencies platform to various committed professionals
placement consultancyacross the globe to use their skills and expertise to join as a recruiter and
interviewer to empower the industry with talented human resources.Searching for the right candidate is never easy.
job consultancy We use crowdsource recruitment to find right talent pool at much faster pace.
Our candidate search follows application of a rigorous methodology, and a comprehensive screening to find an individual
whorecruitment consultants is not only skilled but is also the right culture fit for your organization.
Our interviewers are best in the industry,staffing agencies being experts from various verticals to judge right
candidate for the job. They interview candidates taking into account primarily defined job specification of our clients and targeting
them for needs of the organization.Thinking about payment?placement agencies Don’t worry, you pay when you hire.
Whether you are a startup or an established enterprise, join our 10x faster recruitment process that reduces your hiring process by 50% and give you
manpower consultancyefficient results.
check our website:http://www.incruiter.com.
very nice and great and very beutifull 파워볼총판
azure course Thanks for sharing such a great information..Its really nice and informative..
Effective blog with a lot of information. I just Shared you the link below for Courses .They really provide good level of training and Placement,I just Had Data Science Classes in this institute , Just Check This Link You can get it more information about the Data Science course.
Java training in chennai | Java training in annanagar | Java training in omr | Java training in porur | Java training in tambaram | Java training in velachery
Thanks for Posting article really very Informative Keep Posting
Data Science Training Course In Chennai | Data Science Training Course In Anna Nagar | Data Science Training Course In OMR | Data Science Training Course In Porur | Data Science Training Course In Tambaram | Data Science Training Course In Velachery
Very good post, keep sending us such informative articles I visit your website on a regular basis.
rakesh yadav advance maths pdf
SSC Result 2020 Published Date & Time by ssc result
ssc result 2020
Education Board of Bangladesh.
Many of You Search For SSC Result Kobe Dibe on Internet
as Well as Facebook. The results of Secondary School Certificate
(SSC)—and its equivalent examinations—for 2020 have been published.
SSC & Dakhil Result 2020 Published Date is Very Important For T
he Students Who Attend The SSC Exam 2020.
wow, great, I was wondering how to cure acne naturally. and found your site by google, learned a lot, now i’m a bit clear. I’ve bookmark your site and also add rss. keep us updated.
data science certification
Good information. Thank you for providing information. Study here how you can get rich. 먹튀
Wow What a Nice and Great Article, Thank You So Much for Giving Us Such a Nice & Helpful Information, please keep writing and publishing these types of helpful articles, I visit your website regularly.
rakesh yadav maths
You rock particularly for the high caliber and results-arranged offer assistance. I won't reconsider to embrace your blog entry to anyone who needs and needs bolster about this region.
Software Testing Training in Chennai | Software Testing Training in Anna Nagar | Software Testing Training in OMR | Software Testing Training in Porur | Software Testing Training in Tambaram | Software Testing Training in Velachery
It's very nice to find out other writers share like minds on some content. This is the case with your article. I really enjoyed this.
SEO Services in Kolkata
Best SEO Services in Kolkata
SEO Company in Kolkata
Best SEO Company in Kolkata
Top SEO Company in Kolkata
Top SEO Services in Kolkata
SEO Services in India
SEO Company in India
Up until now, I concur with you on a significant part of the data you have composed here. I should think some on it, yet generally speaking this is a brilliant article.
Online Teaching Platforms
Online Live Class Platform
Online Classroom Platforms
Online Training Platforms
Online Class Software
Virtual Classroom Software
Online Classroom Software
Learning Management System
Learning Management System for Schools
Learning Management System for Colleges
Learning Management System for Universities
I believe there are many more pleasurable opportunities ahead for individuals that looked at your site.thanks a lot guys.
Ai & Artificial Intelligence Course in Chennai
PHP Training in Chennai
Ethical Hacking Course in Chennai Blue Prism Training in Chennai
UiPath Training in Chennai
I found your article on Google when I was surfing, it is written very nicely and is optimized.Thank you I visit your website regularly.
best beaches in goa
This article is packed full of constructive information. The valuable points made here are apparent, brief, clear and poignant.
SAP training in Mumbai
Best SAP training in Mumbai
SAP training institute Mumbai
Thank you for such a nice article keep posting, I am a RegularVisitor of your website.
connaught place
I will be interested in more similar topics. i see you got really very useful topics , i will be always checking your blog thanks
data scientist course malaysia
What an incredible article!. I am bookmarking it to peruse it over again after work. It appears to be an extremely fascinating theme to expound on.
Denial management software
Denials management software
Hospital denial management software
Self Pay Medicaid Insurance Discovery
Uninsured Medicaid Insurance Discovery
Medical billing Denial Management Software
Self Pay to Medicaid
Charity Care Software
Patient Payment Estimator
Underpayment Analyzer
Claim Status
We'll start with a favorite of the upcoming season, the popular pixie haircut. Stylish Pixie. It suits many women. Young girls and ladies over 40 choose it. The secret ...
Haircut Names With Pictures For Ladies
thestyledare.com/
I recently came across your article and have been reading along. I want to express my admiration of your writing skill and ability to make readers read from the beginning to the end. I would like to read newer posts and to share my thoughts with you.
Microsoft Azure certification Online Training in bangalore
Microsoft Azure certification courses in bangalore
Microsoft Azure certification classes in bangalore
Microsoft Azure certification Online Training institute in bangalore
Microsoft Azure certification course syllabus
best Microsoft Azure certification Online Training
Microsoft Azure certification Online Training centers
Such a very useful article. Very interesting to read this article.I would like to thank you for the efforts you had made for writing this awesome article.
SAP Online Training
SAP Classes Online
SAP Training Online
Online SAP Course
SAP Course Online
Very interesting blog Thank you for sharing such a nice and interesting blog and really very helpful article.
Snowflake Online Training
Snowflake Classes Online
Snowflake Training Online
Online Snowflake Course
Snowflake Course Online
Nice article. Thank you for sharing valuable information.
Digital Marketing Training in Chennai | Certification | SEO Training Course | Digital Marketing Training in Bangalore | Certification | SEO Training Course | Digital Marketing Training in Hyderabad | Certification | SEO Training Course | Digital Marketing Training in Coimbatore | Certification | SEO Training Course | Digital Marketing Online Training | Certification | SEO Online Training Course
The article is very interesting and very understood to be read, may be useful for the people. I wanted to thank you for this great read!! I definitely enjoyed every little bit of it. I have to bookmarked to check out new stuff on your post. Thanks for sharing the information keep updating, looking forward for more posts..
Data Science Training In Chennai | Certification | Data Science Courses in Chennai | Data Science Training In Bangalore | Certification | Data Science Courses in Bangalore | Data Science Training In Hyderabad | Certification | Data Science Courses in hyderabad | Data Science Training In Coimbatore | Certification | Data Science Courses in Coimbatore | Data Science Training | Certification | Data Science Online Training Course
Calculate your EMI for personal loan, home loan, car loan, student loan, business loan in India. Check EMI eligibilty,
interest rates, application process, loan.
EMI Calculator calculate EMI for home loan, car loan, personal loan , student loan in India .
visit https://emi-calculators.com/ here for more information.
ترفند برد و آموزش بازی انفجار آنلاین و شرطی، نیترو بهترین و پرمخاطب ترین سایت انفجار ایرانی، نحوه برد و واقعیت ربات ها و هک بازی انجار در
اینجا بخوانید
کازینو آنلاین نیترو
بازی حکم آنلاین نیترو
کازینو آنلاین
بازی حکم آنلاین
Introducing the Nitro Blast game site
معرفی سایت بازی انفجار نیترو
همان طور که می دانید بازی های کازینو های امروزه از محبوبیت ویژه ای برخودارند که این محبوبیت را مدیون سایت های شرط می باشند. با گسترش اینترنت این بازی ها محدودیت های مکانی و زمانی را پشت سرگذاشته و به صورت آنلاین درآمده اند.
بازی انفجار نیترو
بازی انفجار
یکی از محبوب ترین بازی های کازینو، بازی انفجار می باشد که ساخته سایت های شرط بندی می باشد و امروزه از طرفداران ویژه ای برخودار است. با گسترش اینترنت سایت های شرط بندی مختلفی ایجاد شده اند که این بازی را به صورت آنلاین ساپورت می کنند. یکی از این سایت ها، سایت معتبر نیترو می باشد. در این مقاله قصد داریم به معرفی
سایت بازی انفجار نیترو بپردازیم.
سایت پیش بینی فوتبال نیتر
سایت پیش بینی فوتبال
بازی رولت نیترو
Visit https://www.wmsociety.org/
here for more information
Truly, this article is really one of the very best in the history of articles. I am a antique ’Article’ collector and I sometimes read some new articles if I find them interesting. And I found this one pretty fascinating and it should go into my collection. Very good work!
AWS training in Chennai
AWS Online Training in Chennai
AWS training in Bangalore
AWS training in Hyderabad
AWS training in Coimbatore
AWS training
arya samaj mandir in faridabad
I was just browsing through the internet looking for some information and came across your blog. I am impressed by the information that you have on this blog. It shows how well you understand this subject. Bookmarked this page, will come back for more.
data science courses
I see some amazingly important and kept up to length of your strength searching for in your on the site
data science course in noida
Generic Latisse : Eyelashes drops 3ml with Bimatoprost 3%
Natural Ways to Encourage eyelashes growth , iBeauty Care offers a wide variety of natural products for skin care
iBeauty Care offers a wide variety of natural products for skin care, eyelashes growth, acne and many other health problems. All products are with clinically proven effects and great therapeutic results.
Visit https://www.ibeauty care.com/home/21-generic-latisse.html here to buy this ar low cost.
or visit The home page https://www.ibeauty-care.com/.
Very interesting to read this article.I would like to thank you for the efforts. I also offer Data Scientist Courses data scientist courses
wow so nicely written i loved it!!
Data science course in Mumbai
I think this is an informative post and it is very beneficial and knowledgeable. Therefore, I would like to thank you for the endeavors that you have made in writing this article. All the content is absolutely well-researched. Thanks. battery reconditioning scam
Great content thanks for sharing this informative blog which provided me technical information keep posting.
Data Science Training in Chennai
Data Science Training in Velachery
Data Science Training in Tambaram
Data Science Training in Porur
Data Science Training in Omr
Data Science Training in Annanagar
This is such an awesome asset, to the point that you are giving and you give it away for nothing.our article has piqued a lot of positive interest. I can see why since you have done such a good job of making it interesting. yoga burn
camscanner app
meitu app
shein app
youku app
sd movies point
uwatchfree
I am really enjoying reading your well written articles. It looks like you spend a lot of effort and time on your blog. I have bookmarked it and I am looking forward to reading new articles. Keep up the good work.
DevOps Training in Chennai
DevOps Course in Chennai
I see the greatest contents on your blog and I extremely love reading them.
Data Science Training in Hyderabad
Excellent blog thanks for sharing the valuable information..it becomes easy to read and easily understand the information.
Jio Phone Mein Photo Edit Kaise Kare
Best sensitivity settings for pubg mobile 2021
New south movie 2021 hindi dubbed download filmywap
Faug game ko download kaise karte hain 2021
Apna Driving licence kaise check kare online
Very Informative blog
MES Jobs
Junior Auditor Jobs
KFC Jobs in Pakistan
Telenor Jobs
Jazz Jobs
ASF Jobs
ANF Jobs
Interesting stuff to read. Keep it up.Adidas showroom in madurai
Woodland showroom in madurai | Skechers showroom in Madurai
Puma showroom in madurai
Well Said, you have furnished the right information that will be useful to anyone at all time. Thanks for sharing your Ideas.
CCIE Training in Bangalore
Best CCIE Training Institutes in Bangalore
It's Very useful and the topic is clear understanding. Keep sharing. Primavera course online | Primavera p6 training online
I respect this article for the very much investigated substance and magnificent wording. I got so included in this material that I couldn't quit perusing. I am awed with your work and aptitude. Much obliged to you to such an extent.homepage
Really great Post, Thanks for the nice & valuable information. Here I have a suggestion that if your looking for the Best Digital Marketing Training in Gurgaon Then Join the 99 Digital Academy. 99 Digital Academy offers an affordable Digital Marketing Training Course in Gurgaon. Enroll Today.
How to use function key without pressing Fn Key The post was originally published on Loudpedia . You can disable the Fn button and use function keys without pressing the Fn key again and again.
Thanks for the Valuable information.Really useful information. Thank you so much for sharing. It will help everyone.
SASVBA is recognized as the best machine learning training in Delhi. Whether you are a project manager, college student, or IT student, Professionals are the best machine learning institute in Delhi, providing the best learning environment, experienced machine learning instructors, and flexible training programs for the entire module.
FOR ORE INFO:
Nice blog post,
Digital Marketing Course with Internshipthe demand for digital marketing and the job openings that are expected to open in the coming days in mind, we make sure that our Digital Marketing course comes with an internship that focuses on making you work on real-time live projects.
i am glad to discover this page
Thanks for such a great post and the review, I am totally impressed! Keep stuff like this coming.
Best Data Science courses in Hyderabad
It's been a pleasure to tell you about a great opportunity this lockdown . Our institution is offering CS executive classes and only for you guys there will be a free CSEET classes. So don't be late to register. For more information contact us or join our website at https://uniqueacademyforcommerce.com/
Infycle Technologies, the best software training institute in Chennai offers Big Data Hadoop training in Chennai for tech professionals and freshers. In addition to Big Data, Infycle also offers other professional courses such as Python, Oracle, Java, Power BI, Digital Marketing, Data Science, etc., which will be trained with 100% practical classes. After the completion of training, the trainees will be sent for placement interviews in the top MNC's. Call 7502633633 to get more info and a free demo. No.1 Big Data Hadoop Training in Chennai | Infycle Technologies
Thanks for amazing content. Thanks!
Data Science Training in Pune
thanks for this amazing information
full stack developer course
full stack developer course in Bangalore
Shop for the latest Redmi mobiles from Helmet Don at the best prices in India. Xiaomi smartphones include Mi Series, Mi Note Series, Redmi Series, Pocophone, Mi Max Series, Mi Mix Series, and the Blackshark.
HelmetDon
MI
redmi-phones
Shop Repair & Buy Mobiles Online At best prices in India only at https://rshop.in/location/karnal-sadar-bazar/
Forex Leverage for Beginners: Trade at the Next Level
How to Trade Forex Successfully for Beginners – Simple StepsWhat is Needed to Start Forex Trading
Forex Market Hours and Sessions in 2021
How to trade forex using the economic calendar
4 Tips On Setting Up A Forex Trading Station
Forex Leverage for Beginners: Trade at the Next Level
Exness Broker Review 2021
Nice post. Thank you to provide us this useful information.
Visit us: RPA Ui Path Online Training
Visit us: Ui Path Course Online
hi thanku so much this information
home1
all information
HI THANKU SO MUCH THIS INFORMATION
cs executive
freecseetvideolectures/
Thanks for sharing nice information....
data analytics training in aurangabad
Thank you for excellent article.You made an article that is interesting.
ai training in aurangabad
360DigiTMG is the top-ranked and the best Data Science Course Training Institute in Hyderabad..
data analytics course in lucknow
Without data analytics, you cannot imagine data science. In this process, data is examined to transform it into a meaningful aspect.
Without data analytics, you cannot imagine data science. In this process, data is examined to transform it into a meaningful aspect.
I think this is the best article today about future technology. Thanks for taking your own time to discuss this topic, I feel happy that my curiosity has increased to learn more about this topic.
Food Processing Business
Fantastic article I ought to say and thanks to the info. Instruction is absolutely a sticky topic. But remains one of the top issues of the time. I love your article and look forward to more.
Rice Milling Technology
One to One Tuition
Broiler Poultry Farming
Dry Cleaning Business
College Courses
Thanks for the post. It was very interesting and meaningful
best jewellery software jewellery accounting software swarnapp software
software for jewellers
Jewellery Girvi Software
thanks for share this amazing article
Jewellery ERP Software Dubai
Jewellery ERP Software Dubai
Very useful and informative.Thanks for the post.
CCNA course in Pune
Nice Blog
Best Web Development Agency USA
Best Web Development Agency USA
Great article! I appreciate you sharing valuable information.
visit:
Mulesoft Training In Hyderabad
Communication between an Android widget and its application typically involves using `BroadcastReceiver`, `AppWidgetManager`, and `PendingIntent`. The widget can send data to the app using a broadcast, and the app can update the widget using `AppWidgetManager`. For instance, if a user interacts with the widget, a broadcast can trigger an update in the app, which in turn can send an updated view back to the widget. This method ensures seamless interaction between the app and widget, even if the app is not actively running.
Data science courses in Pune
This post on communication between Android widgets and activities is very helpful! The step-by-step guide makes it easier to implement this feature. Thanks for sharing such a clear and practical tutorial!
Data science Courses in Canada
Android widgets enable quick access to app features, like toggling Wi-Fi or GPS on the power control widget. Designing a widget involves planning a seamless communication model with the main application. This ensures efficient interaction and enhances user experience.
Data science Courses in City of Westminster
Neel KBH
kbhneel@gmail.com
Great blog! Thank you for the information.
Data science Courses in Berlin
A brilliant post detailing communication techniques between Android widgets. A fantastic guide for mobile app developers!
Data science courses in France
Post a Comment